Conquering Bootstrap Challenges: A Guide to Mastering the Framework
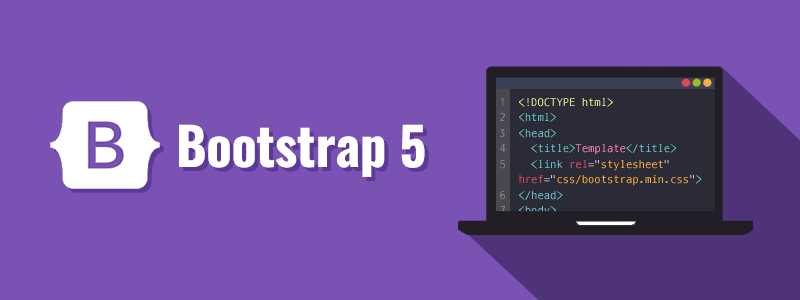
Introduction:
Bootstrap is a popular front-end framework that streamlines web development by providing a robust set of pre-built components and styling options. While Bootstrap offers numerous benefits, it's not uncommon to encounter challenges when working with it. In this blog post, we will explore common Bootstrap challenges and discuss effective strategies for tackling them, helping you become a proficient Bootstrap developer.
Understanding the Bootstrap Framework:
Before delving into the challenges, it's crucial to have a solid understanding of the Bootstrap framework's key components:
1. Grid System:
Bootstrap's responsive grid system allows for creating flexible and responsive layouts. The grid is divided into 12 columns, and developers can leverage the predefined classes to structure their content effectively.
Example:
To create a basic two-column layout, you can use the classes `col-md-6` for each column to distribute the width evenly. You can also utilize responsive breakpoints such as `col-sm` or `col-lg` to define different column widths based on screen sizes.
Bootstrap Documentation: Grid System
2. Components:
Bootstrap provides a wide range of pre-styled components, such as navigation bars, buttons, forms, and cards. These components are designed to be customizable and reusable, offering a streamlined approach to web development.
Example:
To create a navigation bar, you can use the `<nav>` element and apply Bootstrap's classes like `navbar` and `navbar-expand-lg`. By adding additional classes and customizing the navigation structure, you can create a responsive and visually appealing navigation bar.
Bootstrap Documentation: Components
3. Utilities and Helpers:
Bootstrap includes utility classes that assist in handling spacing, alignment, typography, and more. These classes provide additional flexibility and ease of use when styling elements.
Example:
You can utilize utility classes like `mt-3` for top margin, `text-center` for center alignment, or `text-muted` for muted text color. These classes can be applied directly to HTML elements to achieve the desired styling without writing custom CSS.
Bootstrap Documentation: Utilities
Common Bootstrap Challenges and Solutions:
1. Customizing Bootstrap Styles:
Challenge:
Sometimes, you may need to customize the default Bootstrap styles to match your project's unique design requirements.
Solution:
Bootstrap allows for customization through various methods. You can either override the default styles using CSS, leverage Bootstrap's Sass variables to modify core settings, or utilize the official Bootstrap customization tool to generate a customized version of the framework.
Example:
To customize the default button styles, you can override the .btn class in your CSS file and define new styles or modify existing ones. You can change properties like background color, border radius, or font size to match your project's design.
<!-- HTML -->
<button class="btn btn-primary custom-btn">Custom Button</button>
/* CSS */
.custom-btn { background-color: green; font-size: 20px; cursor: pointer; border-radius: 10px; }
In this example, we override the default styles of the Bootstrap button by adding a custom CSS class `.custom-btn` and modifying properties like `background-color`, `font-size`, `cursor` and `border-radius`.
Bootstrap Documentation: Customize
2. Responsiveness and Grid Alignment:
Challenge:
Achieving precise alignment and responsiveness within Bootstrap's grid system can be challenging, especially when working with complex layouts.
Solution:
To address this challenge, understanding how the grid system works is crucial. Utilize the appropriate grid classes, such as `col-md` or `col-lg`, to define column widths based on the target device breakpoints. Experiment with nested grids and offset classes to fine-tune alignments and achieve the desired responsive behavior.
Additionally, take advantage of Bootstrap's responsive utility classes, such as `d-none` and `d-flex`, to control the visibility and behavior of elements on different screen sizes. Use media queries to apply custom styles and adjust layouts for specific breakpoints, ensuring a seamless user experience across devices.
Example:
Suppose you want to create a three-column layout on large screens and a single-column layout on smaller screens. You can use classes like col-lg-4 for each column on large screens and col-12 for single-column layout on smaller screens.
<!-- HTML -->
<div class="container"> <div class="row"> <div class="col-lg-4 col-md-3"> Column 1</div> <div class="col-lg-4 col-md-6"> Column 2</div> <div class="col-lg-4 col-md-12"> Column 3</div> </div> </div>
In this example, we use different grid classes to define column widths for different screen sizes. The columns will stack vertically on smaller screens (col-md-3, col-md-6 and col-md-12) and appear side by side on larger screens (col-lg-4).
Bootstrap Documentation: Grid System
3. Integration of Custom JavaScript Functionality:
Challenge:
At times, you may need to integrate custom JavaScript functionality or third-party plugins into your Bootstrap project, which can pose challenges due to conflicts or compatibility issues.
Solution:
Ensure that you carefully read the documentation and follow the guidelines provided by Bootstrap and the third-party plugin. Pay attention to any potential conflicts between JavaScript libraries and resolve them by using appropriate namespacing or jQuery noConflict mode. Be diligent in testing and debugging to ensure smooth integration of custom JavaScript functionality.
To mitigate conflicts, consider using package managers like npm or yarn to manage dependencies effectively. These tools allow you to specify version ranges, ensuring compatibility between Bootstrap and other libraries.
Example:
If you want to integrate a datepicker plugin into your Bootstrap project, you would need to follow the plugin's documentation for installation and usage instructions. You may need to load the necessary JavaScript files, initialize the plugin with appropriate options, and handle any event callbacks.
<!-- HTML -->
<div id="myElement"> Content to be modified </div>
<!-- JavaScript -->
<script src="jquery.js"></script> <script src="bootstrap.js"></script> <script> $(document).ready(function() {
// Custom JavaScript functionality
$('#myElement').addClass('show'); }); </script>
In this example, we integrate custom JavaScript functionality using jQuery. We add a class "show" to the element with the id "myElement" when the document is ready. Ensure that you include the necessary JavaScript files, such as jQuery and Bootstrap, before your custom script.
Bootstrap Documentation: JavaScript
4. Managing Bootstrap Updates:
Challenge:
Bootstrap releases updates periodically, introducing new features, bug fixes, and improvements. Managing updates without disrupting existing projects can be a challenge.
Solution:
Before updating Bootstrap, thoroughly review the release notes and documentation to understand the changes and potential impacts on your project. Create a backup of your project files and gradually implement the updates in a testing environment. Test the updated components and styles thoroughly to identify any compatibility issues and make necessary adjustments before deploying the changes to production.
Adopt version control systems like Git to track changes and easily roll back to a previous version if necessary. Utilize package managers or CDNs to ensure seamless updates without manually modifying and replacing Bootstrap files in your projects.
Example:
Suppose you are currently using Bootstrap 4 in your project and want to update to Bootstrap 5. You would need to review the migration guide provided by Bootstrap to understand the necessary changes and considerations. Then, you can update the Bootstrap files in your project, test the affected components and styles, and make any required modifications.
Bootstrap Documentation: Migration
5. Overcoming Performance Concerns:
Challenge:
Including the entire Bootstrap framework can lead to larger file sizes, affecting page load times and performance.
Solution:
Optimize Bootstrap by customizing and selectively including only the required components and styles for your project. Utilize tools like Bootstrap's official build tools or task runners like Grunt or Gulp to minimize and concatenate CSS and JavaScript files, reducing the overall file size. Implement browser caching and compression techniques to further enhance performance.
Consider utilizing content delivery networks (CDNs) to serve Bootstrap files, taking advantage of caching and distributed servers to optimize load times. Optimize and compress images used within Bootstrap components to minimize their impact on page performance.
<!-- JavaScript -->
module.exports = function(grunt) { grunt.initConfig({ concat: { options: { separator: '\n\n', }, dist: { src: ['bootstrap.css', 'custom.css'], dest: 'dist/styles.min.css', }, }, uglify: { options: { compress: true, }, dist: { src: ['bootstrap.js', 'custom.js'], dest: 'dist/scripts.min.js', }, }, }); grunt.loadNpmTasks('grunt-contrib-concat'); grunt.loadNpmTasks('grunt-contrib-uglify'); grunt.registerTask('default', ['concat', 'uglify']); };
This Grunt configuration concatenates and minifies the CSS files (bootstrap.css and custom.css) into `dist/styles.min.css` and the JS files (bootstrap.js and custom.js) into `dist/scripts.min.js`.
These code snippets provide a basic understanding and implementation of the examples mentioned. Feel free to modify them according to your specific needs and project requirements.
Remember to include the necessary libraries and dependencies based on your project setup, such as jQuery, Bootstrap, and Grunt or any other build tools you prefer.
Example:
Instead of including the entire Bootstrap CSS and JavaScript files, you can use the official customization tool to select only the components you need. This reduces the file size and improves the performance of your website.
Bootstrap Documentation: Optimization
Best Practices for Working with Bootstrap:
To overcome Bootstrap challenges more effectively, consider adopting the following best practices:
1. Stay Up-to-Date:
Keep yourself informed about the latest Bootstrap updates, documentation, and community resources. Regularly visit the Bootstrap website and join relevant forums or communities to stay connected with fellow developers and learn from their experiences.
2. Plan and Prototype:
Before diving into development, plan your project's structure and layout. Create wireframes or prototypes to visualize how the Bootstrap components will fit into your design. This upfront planning can save time and help you anticipate potential challenges.
3. Leverage Documentation:
Bootstrap provides comprehensive documentation that covers each component, its usage, and customization options. Familiarize yourself with the documentation and refer to it whenever you encounter challenges or have questions.
4. Experiment and Practice:
Bootstrap mastery comes with practice. Set aside time to experiment with different components, layouts, and customization options. Build small projects or participate in coding challenges to enhance your skills and gain confidence in working with Bootstrap.
5. Seek Community Support:
The Bootstrap community is vast and supportive. Engage with other developers through forums, social media groups, or developer communities. Sharing your challenges and seeking advice from experienced developers can provide valuable insights and solutions.
Conclusion:
Working with Bootstrap offers numerous benefits but can present certain challenges along the way. By understanding the framework's core components, adopting effective strategies to overcome challenges, and following best practices, you can become a proficient Bootstrap developer. Embrace continuous learning, experimentation, and collaboration within the Bootstrap community to enhance your skills and create exceptional web experiences using this powerful front-end framework.