Understanding Behavior-Driven Development (BDD) Testing
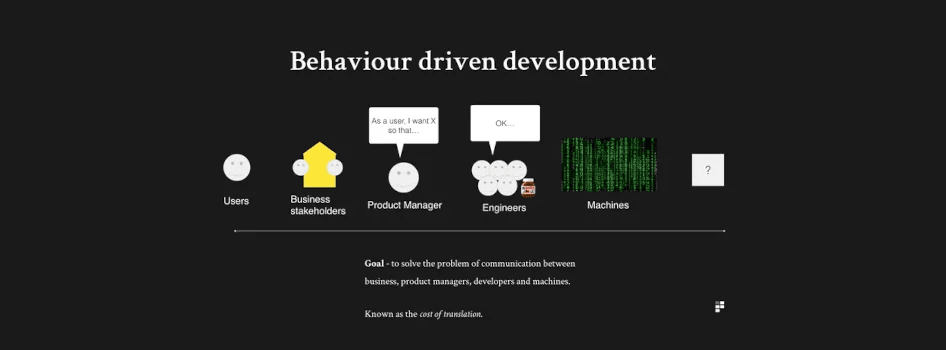
Overview -
In the world of software development, testing is an essential process to ensure that your application functions as expected and meets the requirements of your users. Behavior-Driven Development (BDD) is an approach that has gained popularity in recent years for its ability to bridge the communication gap between developers, testers, and non-technical stakeholders while ensuring effective and comprehensive testing. In this blog post, we will delve into what BDD testing is, its principles, benefits, and how to get started with it.
What is BDD Testing?
Behavior-Driven Development (BDD) is an agile software development methodology that focuses on the behavior of a software application from the user's perspective. BDD testing extends this methodology by emphasizing collaboration between different roles within a development team, such as developers, testers, product owners, and business analysts, to create a shared understanding of the expected behavior of the software.
At its core, BDD testing involves writing test cases in plain, human-readable language, often referred to as "Gherkin" language, which is easy for both technical and non-technical team members to understand. These test cases describe the desired behavior of the software through scenarios that outline the expected interactions and outcomes.
Key Principles of BDD Testing
1. Collaboration: BDD encourages collaboration among team members to define and understand the desired behavior of the software. This collaboration helps ensure that everyone involved has a shared understanding of the application's requirements.
2. User-Centric: BDD focuses on the user's perspective, emphasizing how the application should behave and meet the needs of the end-users.
3. Gherkin Language: BDD test cases are written in plain language using the Gherkin syntax, which consists of keywords like "Given," "When," and "Then" to describe the initial context, actions, and expected outcomes of a scenario.
4. Automation: While BDD tests start as human-readable scenarios, they can be automated using testing frameworks like Cucumber, SpecFlow, or Behave. This allows for the efficient execution of tests and integration with the development workflow.
Benefits of BDD Testing
Implementing BDD testing in your software development process can yield several benefits:
1. Improved Communication: BDD encourages open and clear communication among team members, reducing misunderstandings and ensuring that everyone is on the same page regarding the software's requirements.
2. Enhanced Test Coverage: BDD tests focus on the behavior of the application, which often results in more comprehensive test coverage compared to traditional testing methods.
3. Documentation: BDD scenarios serve as living documentation that can be easily maintained and updated as the software evolves. This documentation aids in onboarding new team members and provides a clear reference for existing ones.
4. Early Detection of Issues: BDD testing allows for the early identification of potential issues and discrepancies between requirements and implementation, reducing the cost of fixing defects later in the development cycle.
5. Increased Reusability: BDD test scenarios can be reused for different test cases and even across projects, saving time and effort in test case creation.
Getting Started with BDD Testing
To start implementing BDD testing in your development process, follow these steps:
1. Select a BDD Framework: Choose a BDD testing framework that aligns with your programming language and technology stack. Popular choices include Cucumber (for Java, Ruby, and more), SpecFlow (for .NET), and Behave (for Python).
2. Define Scenarios: Collaborate with your team to define scenarios that describe the desired behavior of the software. Use the Gherkin language to write these scenarios in a structured format.
3. Automate Tests: Write step definitions or bindings for your scenarios using the chosen BDD framework. These step definitions map the Gherkin language to executable code, allowing you to automate your tests
4. Execute Tests: Run your BDD tests regularly as part of your continuous integration (CI) pipeline or during manual testing. Ensure that your tests provide feedback on the application's behavior.
5. Iterate and Refine: Continuously update and refine your BDD scenarios and step definitions as the software evolves and new requirements emerge.
Tools we use for BDD in gai
Incorporating BDD tools into your software development process can significantly improve the quality and reliability of your applications. These tools enable teams to collaborate effectively, write human-readable test scenarios, and automate testing, leading to better communication and fewer defects. Whether you're working with Java, Python, .NET, or other programming languages, there's a BDD tool available to suit your needs. Choose the one that aligns with your project requirements and start reaping the benefits of Behavior-Driven Development in your testing efforts.
1. Cucumber
Cucumber is one of the most popular BDD tools, known for its versatility and support for multiple programming languages like Java, Ruby, JavaScript, and more. It uses the Gherkin language to write human-readable test scenarios. These scenarios can be executed against the application's code, allowing teams to automate tests easily. Cucumber promotes collaboration among team members by providing a common language for describing and verifying behavior.
Key Features:
- Support for various programming languages.
- Integration with popular testing frameworks.
- Extensive documentation and community support.
- Detailed test reports for easy analysis.
2. JBehave (Java)
JBehave is a BDD framework for Java applications. It encourages collaboration through the use of natural language scenarios and Java step definitions. JBehave supports the creation of reusable and maintainable test scripts, making it suitable for large-scale projects. It offers integration with popular Java testing tools like JUnit and TestNG.
Key Features:
- Native support for Java.
- Integration with Java testing frameworks.
- Hierarchical story and scenario organization.
- Robust reporting and visualization capabilities.
Sample:
Here's an example of a BDD test using Java and the Cucumber framework. In this example, we'll test a simple login functionality of a web application.
1. First, you'll need to set up your Java project with Cucumber. You can use tools like Maven or Gradle to manage your dependencies.
2. Create a feature file named `login.feature` with the following content:
```gherkin
Feature: User Login
Scenario: Valid login
Given the user is on the login page
When the user enters valid credentials
And clicks the login button
Then they should be redirected to the dashboard
Scenario: Invalid login
Given the user is on the login page
When the user enters invalid credentials
And clicks the login button
Then an error message should be displayed
In this example:
- "Feature" describes the high-level feature you're testing, in this case, addition.
- "Scenario" defines specific test cases within the feature, each with a given-when-then structure.
- "Given" represents the initial context or setup.
- "When" describes the action or event being tested.
- "Then" specifies the expected outcome or result.
3. Create step definitions for your feature file. Create a Java class, for example, `LoginSteps.java`, and define the step definitions like this:
```java import io.cucumber.java.en.Given; import io.cucumber.java.en.When; import io.cucumber.java.en.Then; public class LoginSteps { @Given("the user is on the login page") public void the_user_is_on_the_login_page() { // Navigate to the login page in your web application } @When("the user enters valid credentials") public void the_user_enters_valid_credentials() { // Enter valid credentials (e.g., username and password) } @When("the user enters invalid credentials") public void the_user_enters_invalid_credentials() { // Enter invalid credentials (e.g., incorrect username or password) } @When("clicks the login button") public void clicks_the_login_button() { // Click the login button on the web page } @Then("they should be redirected to the dashboard") public void they_should_be_redirected_to_the_dashboard() { // Verify that the user is redirected to the dashboard page } @Then("an error message should be displayed") public void an_error_message_should_be_displayed() { // Verify that an error message is displayed on the login page } }
4. Finally, you need to configure Cucumber to run your tests. You can use a JUnit runner or other methods provided by Cucumber to execute your tests. Here's a simple JUnit runner class:
```java import io.cucumber.junit.Cucumber; import io.cucumber.junit.CucumberOptions; import org.junit.runner.RunWith; @RunWith(Cucumber.class) @CucumberOptions( features = "src/test/resources/features", glue = "your.package.name", // Replace with the package where your step definitions are located plugin = {"pretty", "html:target/cucumber-reports"} ) public class RunCucumberTests { }
Make sure to replace `"your.package.name"` with the actual package where your `LoginSteps` class is located.
5. Run your tests using JUnit, and Cucumber will execute the scenarios defined in your feature file using the step definitions you've provided.
This is a basic example of BDD using Java and Cucumber. You can expand and customize it to test more complex functionality in your application.
Conclusion-
Behavior-Driven Development (BDD) testing is a powerful approach that not only improves the quality of your software but also fosters collaboration and shared understanding among team members. By focusing on the behavior of your application from the user's perspective and using plain language scenarios, BDD testing helps bridge the gap between technical and non-technical stakeholders. As you adopt BDD testing, you'll find that it not only enhances your testing process but also contributes to the overall success of your software development projects.